Recovering saved wifi networks from my old Huawei Smartphone
I recently got a new smartphone, but have been unable to find a way to transfer the saved Wi-Fi networks from my old phone to my new one.
All the online tutorials just say "go to settings and generate a QR code" but it just does not work. The Huawei Android only allows you to generate a QR code for the network you are currently connected to (yes, I could run to each of the wifi networks, pull out my old phone, generate a QR and scan it with the new one, but that is just stupid).
All the other alternatives involve rooting the phone, which is quite complicated on some Huawei phones (requires ripping off the glass back, etc).
So this is what finally worked:
Android SDK Platform Tools
Sounds like a huge pile of software but it is actually quite harmless. Download the portable zip from here (or google "adb android download") and extract it.
Open the folder and inside Rightclick > Open in terminal, to have the windows terminal or powershell open and navigated into the folder.
Connect your phone using USB to the computer, you also need to enable usb debugging in the developer tools (plenty of tutorials online)
Now we do a backup using
.\adb.exe backup -f backup.ab -shared -all -system -keyvalue
In my case I was prompted to unlock the phone and confirm on the screen. Do not choose a password. This should take a bit and then generate a "backup.ab" file
Android Backup extractor
Download the latest jar from here and put it in the folder. You obviously need java to execute it so download and install that too (you might need to restart your terminal if the java
command does not work)
Now run
java -jar .\abe.jar unpack .\backup.ab backup.tar
(your jar file might have a different name) to decrypt the backup into the backup.tar
This is a file you can open using any archive tool like 7Zip or WinRAR.
Search for the file backup.tar>_/apps/com.android.providers.settings/k/com.android.providers.settings.data
This contains all ssids and their password but we can continue to have a more human-readable format
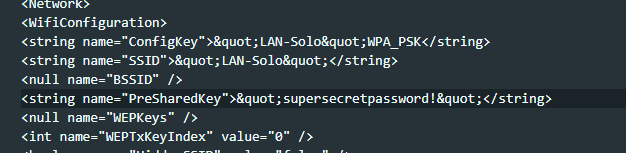
Little script magic
There are many ways to do this but as you might know me I choose node.js
const fs = require('fs');
const settingsData = fs.readFileSync('com.android.providers.settings.data', 'utf8');
const regex = /<string name="SSID">"(.*?)"<\/string>[\s\S]*?(?:<string name="PreSharedKey">"(.*?)"<\/string>|<null name="PreSharedKey" \/>)/gm;
const matches = [...settingsData.matchAll(regex)];
const wifi = matches.map(match => ({ SSID: match[1], Key: match[2] }));
wifi.sort((a, b) => a.SSID.toLowerCase().localeCompare(b.SSID.toLowerCase()));
console.log(wifi);
Or even a little more fancy to just generate qr codes
const qr = require('qrcode-terminal');
.....
wifi.forEach(network => {
if (network.Key) {
const wifiString = `WIFI:T:WPA;S:${network.SSID};P:${network.Key};;`;
console.log(`\nQR Code for SSID: ${network.SSID}`);
qr.generate(wifiString, { small: true });
}
});